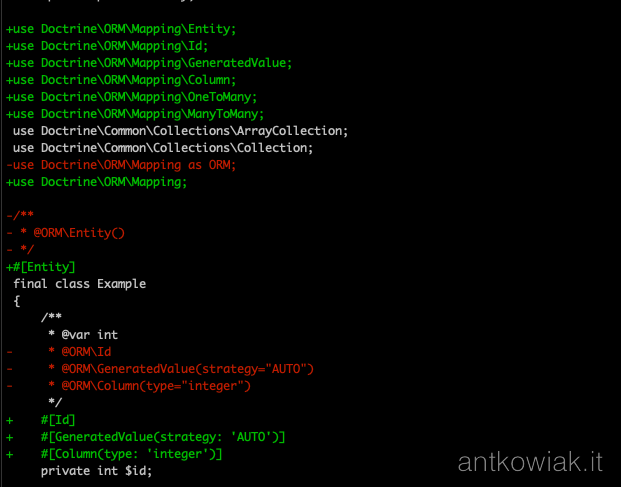
PHP as of version 8.0 has native attributes. To easily convert annotations into attributes throughout the application, we can use the Rector .
Where can we use attributes instead of annotations?
- Doctrine entites mapping
- Routing mapping
- All custom annotations that we wrote
- Validator configuration
What will we need?
- Rector
To install rector type in the console:
composer require rector/rector --dev
- Doctrine version
>=2.9
After installing the rector and making sure you have doctrine version >=2.9, initialize the rector using the command
vendor/bin/rector init
The rector.php
configuration file should appear in the root directory.
Below the configuration to turn annotations into attributes for an application written in Symfony.
<?php
declare(strict_types=1);
use Rector\CodingStyle\Rector\Use_\RemoveUnusedAliasRector;
use Rector\Core\Configuration\Option;
use Rector\Doctrine\Set\DoctrineSetList;
use Rector\Symfony\Set\SymfonySetList;
use Symfony\Component\DependencyInjection\Loader\Configurator\ContainerConfigurator;
return static function (ContainerConfigurator $containerConfigurator): void {
$parameters = $containerConfigurator->parameters();
$parameters->set(Option::AUTO_IMPORT_NAMES, true);
$parameters->set(Option::IMPORT_SHORT_CLASSES, true);
$containerConfigurator->import(DoctrineSetList::ANNOTATIONS_TO_ATTRIBUTES);
$containerConfigurator->import(SymfonySetList::ANNOTATIONS_TO_ATTRIBUTES);
$containerConfigurator->import(SymfonySetList::SYMFONY_CODE_QUALITY);
$containerConfigurator->import(SymfonySetList::SYMFONY_CONSTRUCTOR_INJECTION);
$services = $containerConfigurator->services();
$services->set(RemoveUnusedAliasRector::class);
};
Then we can check what changes the rector will make to the files
vendor/bin/rector process src --dry-run
If you don’t notice anything alarming, just use the above command without --dry-run
vendor/bin/rector process src
At the end we have to change the doctrine.yaml
configuration file, we are looking for the parameter responsible for the mapping type, it is located in doctrine.orm.mappings.{namespace[default App]}.type
and we replace annotation
with attribute
.
That’s all, at the end if we don’t need the rector just remove it:
composer remove rector/rector --dev
Then delete the rector.php
file.